Strings
A string is a sequence of characters. Python treats anything inside quotes as a string. This includes letters, numbers, and symbols. Python has no character data type so single character is a string of length 1.
s1 = "Hello"
s2 = 'wor'd'
print(s1,s2)
Multi line string
s = """I am Learning
Python String"""
print(s)
Accessing characters in Python String
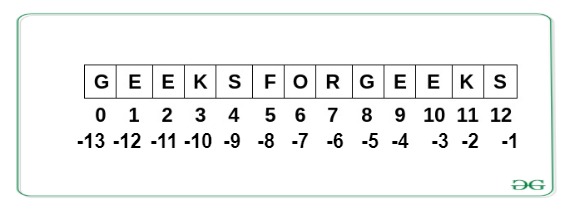
s = "Hello World"
# Accesses first character: 'H'
print(s[0])
# Accesses 5th character: 'o'
print(s[4])
print(s[-1])
print(s[-2])
String Slicing
Slicing is a way to extract portion of a string by specifying the start and end indexes. The syntax for slicing is string[start:end], where start starting index and end is stopping index (excluded).
s = "Hello World"
# Retrieves characters from index 1 to 3: 'ell'
print(s[1:4])
# Retrieves characters from beginning to index 2: 'Hel'
print(s[:3])
# Retrieves characters from index 3 to the end: 'lo World'
print(s[3:])
# Reverse a string 'dlrow olleh'
print(s[::-1])
String Immutability
# Trying to change the first character raises an error
# s[0] = ''H" # Uncommenting this line will cause a TypeError
# Instead, create a new string
s = "H" + s[1:]
print(s)
Sample Code
s = "hello world"
# Updating by creating a new string
s1 = "H" + s[1:]
# replacnig "world" with "pythonworld"
s2 = s.replace("world", "python world")
print(s1)
print(s2)
# Length of string
x = len(s)
print("length of s if ",x)
# Upper case
y = s.upper()
print("upper case: ",y)
# Lower case
z = s.lower()
print("lower case: ",z)
# remove empty spaces
str1 = "Hello w o r l d"
str2 = str1.strip()
print("strip: ",str2)
# Concat
str3 = str1 + " " + str2
# multiple
print(str1 * 3)
# find
s = "Hello World"
print("ello" in s)
print("HWH" in s)