Variables in Python
Variables act as placeholders for data. They allow us to store and reuse values in our program.
# Variable 'x' stores the integer value 5
x = 5
# Variable 'name' stores the string "Sam"
name = "Sam"
Rules for Naming Variables
To use variables effectively, we must follow Python’s naming rules:
- Variable names can only contain letters, digits and underscores (_).
- A variable name cannot start with a digit.
- Variable names are case-sensitive (myVar and myvar are different).
- Avoid using Python keywords (e.g., if, else, for) as variable names.
Examples
Valid Example
age = 21
_colour = "lilac"
total_score = 90
Invalid example
1name = "Error" # Starts with a digit
class = 10 # 'class' is a reserved keyword
user-name = "sam" # Contains a hyphen
Basic Assigment
x = 5
y = 3.14
z = "Hi"
Dynamic Typing
x = 10
print(type(x))
x = "Now a string"
print(type(x))
Multiple Assignments
a = b = c = 100
print(a, b, c)
x, y, z = 1, 2.5, "Python"
print(x, y, z)
Type casting
Casting refers to the process of converting the value of one data type into another. Python provides several built-in functions to facilitate casting, including int(), float() and str() among others.
Basic Casting Functions
- int() – Converts compatible values to an integer.
- float() – Transforms values into floating-point numbers.
- str() – Converts any data type into a string
# Casting variables
s = "10" # Initially a string
n = int(s) # Cast string to integer
cnt = 5
f = float(cnt) # Cast integer to float
age = 25
s2 = str(age) # Cast integer to string
# Display results
print(n)
print(cnt)
print(s2)
Getting the Type of Variable
In Python, we can determine the type of a variable using the type() function. This built-in function returns the type of the object passed to it.
n = 42
print(type(n))
Scope of Variables
local Variables
Variables defined inside a function are local to that function.
def f():
a = "I am local"
print(a)
f()
# print(a)
# This would raise an error since 'local_var' is not accessible outside the function
Global Variables
Variables defined outside any function are global and can be accessed inside functions using the global keyword.
a = "I am global"
def f():
global b
b = "b is also globally available"
print(a)
f()
print(b)
Undertstanding assigment
x = 5
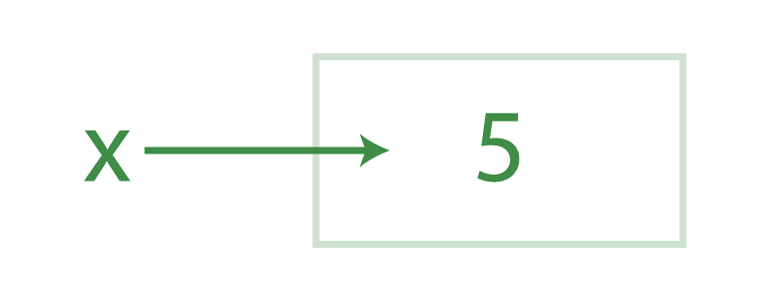
Now, if we assign another variable y to the variable x.
y = x
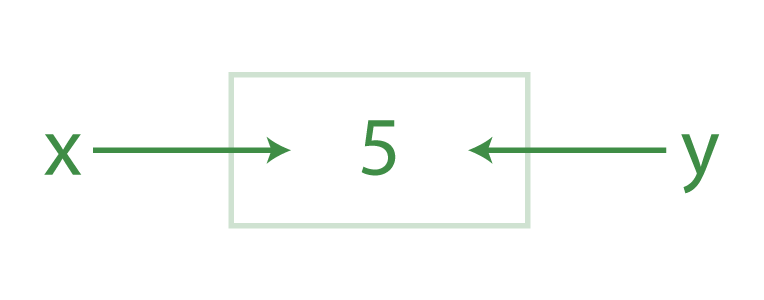
del variable
We can remove a variable from the namespace using the del keyword. This effectively deletes the variable and frees up the memory it was using.
# Assigning value to variable
x = 10
print(x)
# Removing the variable using del
del x
# Trying to print x after deletion will raise an error
# print(x) # Uncommenting this line will raise NameError: name 'x' is not defined